Cpp.js
Bind C++ to JavaScript with no extra code
- Prerequisites
- npm
- pnpm
- yarn
- bun
ALL: Node.js >= 18, Docker MOBILE: CMake >= 3.28 IOS: Xcode, Cocoapods
npm create cpp.js@latest
pnpm create cpp.js@latest
yarn create cpp.js@latest
bun create cpp.js@latest
- C++ & JS (Cpp.js)
- JS Only
- Run in Browser
import { initCppJs, Matrix } from './native/Matrix.h';
await initCppJs();
const a = new Matrix(1210000, 1);
const b = new Matrix(1210000, 2);
const result = a.multiple(b);
console.log(result.get(0)); // execution time: 0.872s
class Matrix : public std::vector<int> {
public:
Matrix(int size, int v) : std::vector<int>(size, v) {}
int get(int i) { return this->at(i); }
std::shared_ptr<Matrix> multiple(std::shared_ptr<Matrix> b) {
int size = sqrt(this->size());
auto result = std::make_shared<Matrix>(this->size(), 0);
for (int i = 0; i < size; i += 1) {
for (int j = 0; j < size; j += 1) {
for (int k = 0; k < size; k += 1) {
(*result)[i*size+j]+=this->at(i*size+k)*(*b)[k*size+j];
}
}
}
return result;
}
};
import { Matrix } from './Matrix.js';
const a = new Matrix(1210000, 1);
const b = new Matrix(1210000, 2);
const result = a.multiple(b);
console.log(result.get(0)); // execution time: 5.886s
export class Matrix extends Array {
constructor(size, v) { super(size); this.fill(v); }
get(i) { return this[i]; }
multiple(otherMatrix) {
const size = Math.sqrt(this.length);
const result = new Matrix(this.length, 0);
for (let i = 0; i < size; i += 1) {
for (let j = 0; j < size; j += 1) {
for (let k = 0; k < size; k += 1) {
result[i*size+j]+=this[i*size+k]*otherMatrix[k*size+j];
}
}
}
return result;
}
}
Features
Inter-Process Communication
Write your frontend in JavaScript and power it with C++ logic. Cpp.js empowers developers to bridge the gap between these two worlds seamlessly and efficiently.
Cpp.js supports binding for most C++ constructs, including features from C++11 and C++14.
- Classes: Constructors, member functions, inheritance, polymorphism, and interfaces
- Functions: Function calls and overloading
- Data Types: Primitive types, vectors, maps, enums, and class objects
Cross Platform
C++ and JavaScript can run on any platform. Cpp.js compiles your C++ code to the relevant platforms, ensuring it runs seamlessly on the web, Android, and iOS.
- WebAssembly: Chrome, Firefox, Safari, Node.js, Cloudflare Workers
- Android: Android arm64 and x86_64
- iOS: iOS arm64 and arm64e for iPhone and iPhone simulator
Prebuilt Libraries
Cpp.js offers a range of precompiled libraries, enabling effortless integration into your projects without the need for manual compilation. These libraries streamline the development process, allowing you to focus on building high-performance applications.
Additionally, users can create and distribute their own precompiled libraries, further enhancing flexibility and collaboration within the developer community.
Compile Existing Projects
Cpp.js can build projects that use CMake or configure and package them into a Cpp.js package. Below is a configuration for building the TIFF library:
export default {
getURL: (version) => `https://download.osgeo.org/libtiff/tiff-${version}.tar.gz`,
buildType: 'cmake',
getBuildParams: () => [
'-Dtiff-tools=OFF', '-Dtiff-tests=OFF', '-Dtiff-contrib=OFF',
'-Dtiff-docs=OFF', '-Dld-version-script=OFF',
],
};
High Performance Execution
Cpp.js is engineered to provide high execution speeds, optimizing performance for applications. By compiling C++ code into WebAssembly for the web and native machine code for mobile, it ensures maximum efficiency and performance.
Cpp.js
JavaScript
Test Url: https://codepen.io/bugra9/pen/qBzvvbZ
Bundler Integration
Cpp.js integrates seamlessly with popular JavaScript bundlers to streamline the development process.
Supports Webpack, Rollup, Vite, Rspack, Create React App (CRA), React Native, Metro, Gradle, Cocoapods.
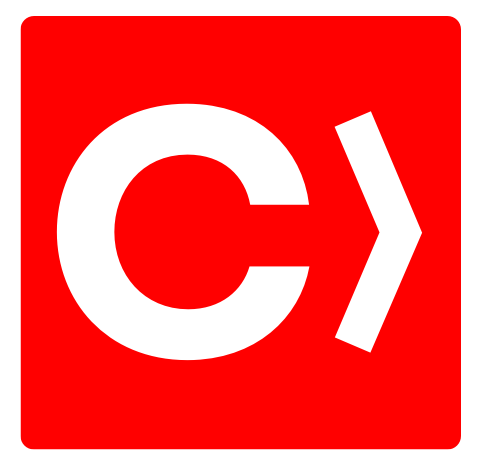